Schedule Flows on AWS Step Functions
Question
How can I schedule flows to run at a specific time on AWS?
Solution
There is a Metaflow decorator for that!
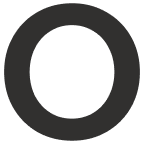
Outerbounds user note
If you run Metaflow on Outerbounds, click here to follow the twin of this guide which uses Argo workflows and does not depend on AWS.
1Scheduling Flows
You can use Metaflow's @schedule
flow-level decorator to run on AWS Step Functions where Metaflow automatically maps a FlowSpec
onto an AWS Step Functions state machine. Alternatively, you can schedule flows with Argo Workflows.
After deploying the script containing your flow to AWS Step Functions the execution of a FlowSpec
can happen on any event-based trigger you setup or a time-based trigger defined with Metaflow's @schedule
decorator.
2Run Flow
This flow is scheduled to run daily. Notice Metaflow's @schedule
decorator has arguments that determine when the flow is run. Time based triggers you can use include:
@schedule(weekly=True)
runs the workflow on Sundays at midnight.@schedule(daily=True)
runs the workflow every day at midnight.@schedule(hourly=True)
runs the workflow every hour.@schedule(cron='0 10 * * ? *')
runs the workflow at the given Cron schedule, in this case at 10am UTC every day. You can use the rules defined here to define the schedule for the cron option.
from metaflow import FlowSpec, schedule, step
from datetime import datetime
@schedule(daily=True)
class DailyFlowAWS(FlowSpec):
@step
def start(self):
now = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
print('time is %s' % now)
self.next(self.end)
@step
def end(self):
pass
if __name__ == '__main__':
DailyFlowAWS()
python schedule_flow_aws.py --with retry step-functions create
After running the above command your flow will be triggered daily!
3Manually Trigger Flow
You can manually trigger the flow at any time:
python schedule_flow_aws.py step-functions trigger
You can also interact with the flow through your AWS Step Functions console: